Discover Erik's code
001using System;002using System.Collections.Generic;003using System.Linq;004using VanRiet.Shipment.Sorter.Weighing.Strategies;005using static VanRiet.Shipment.Sorter.Weighing.Constants;006007namespace VanRiet.Shipment.Sorter.Weighing008{009 public class WeightAllowanceCalculator010 {011 public virtual WeightAllowanceCalculationResult Calculate(uint weightInGrams)012 {013 var strategy = new PreCalculatedWeightStrategy(weightInGrams);014015 return CalculateWeightAllowance(strategy);016 }017018 public virtual WeightAllowanceCalculationResult Calculate(019 WeightAllowanceCalculationInformation info)020 {021 var strategy = ChooseStrategy();022023 return CalculateWeightAllowance(strategy);024025 IShipmentWeightAllowanceStrategy ChooseStrategy()026 {027 if (!info.Products.Any())028 return new UnknownShipmentStrategy();029030 var anyProductsWithUnknownWeight =031 info.Products.Any(p => !p.WeightGrams.HasValue);032033 if (anyProductsWithUnknownWeight && info.IsMultiColli)034 return new UnknownShipmentStrategy();035036 if (info.IsMultiColli)037 return new MultiColliShipmentStrategy(info);038039 if (anyProductsWithUnknownWeight)040 return new UnknownProductWeightStrategy(info);041042 if (info.PreCalculatedWeight.HasValue)043 return new PreCalculatedWeightStrategy(info.PreCalculatedWeight.Value);044045 return new SingleShipmentStrategy(info);046 }047 }048049 private WeightAllowanceCalculationResult CalculateWeightAllowance(050 IShipmentWeightAllowanceStrategy input051 )052 {053 checked054 {055 var intermediateResults = new List<WeightAllowanceCorrection>();056057 var result = ApplyToleranceCoefficientsToProductsWeight();058 result = ApplyMaximumAllowedProductsWeightCorrection(result);059 result = AddPackaging(result);060 result = ApplySupportedGrossWeightCorrection(result);061 result = ApplySensorsAccuracyCorrection(result);062063 return new WeightAllowanceCalculationResult(064 new WeightRangeGrams(065 min: (uint) result.min,066 max: (uint) result.max067 ),068 input.PackagingType,069 input.GetType(),070 intermediateResults071 );072073 (decimal min, decimal max) ApplyToleranceCoefficientsToProductsWeight()074 {075 decimal calculatedMin = Math.Floor(076 input.Products.Min * _settings.MinimumProductWeightToleranceCoefficient077 );078079 decimal calculatedMax = Math.Ceiling(080 input.Products.Max * _settings.MaximumProductWeightToleranceCoefficient081 );082083 var correctedRange = (calculatedMin, calculatedMax);084085 AddIntermediateResult(086 WeightAllowanceCorrectionType.AppliedProductWeightToleranceCoefficients,087 original: (input.Products.Min, input.Products.Max),088 corrected: correctedRange089 );090091 return correctedRange;092 }093094 void AddIntermediateResult(WeightAllowanceCorrectionType type, (decimal, decimal) original, (decimal, decimal) corrected) =>095 intermediateResults.Add(new WeightAllowanceCorrection(type, original: original, corrected: corrected));096097 (decimal min, decimal max) AddPackaging((decimal min, decimal max) range)098 {099 var correctedRange = (range.min + input.Packaging.Min, range.max + input.Packaging.Max);100101 AddIntermediateResult(102 WeightAllowanceCorrectionType.AddedPackagingWeight,103 original: range,104 corrected: correctedRange105 );106107 return correctedRange;108 }109110 (decimal min, decimal max) ApplySensorsAccuracyCorrection(111 (decimal min, decimal max) range112 )113 {114 decimal calculatedMin = Math.Max(range.min - SensorsAccuracyGrams, 0);115 decimal calculatedMax = range.max + SensorsAccuracyGrams;116117 var correctedRange = (calculatedMin, calculatedMax);118119 AddIntermediateResult(120 WeightAllowanceCorrectionType.IncludedSensorsAccuracy,121 original: range,122 corrected: correctedRange123 );124125 return correctedRange;126 }127128 (decimal min, decimal max) ApplyMaximumAllowedProductsWeightCorrection(129 (decimal min, decimal max) range130 )131 {132 decimal calculatedMin = range.min;133 decimal calculatedMax = range.max;134 calculatedMin = Math.Min(calculatedMin, input.MaxSupportedWeightGrams);135 calculatedMax = Math.Min(calculatedMax, input.MaxSupportedWeightGrams);136137 var corrected = (calculatedMin, calculatedMax);138139 if (range != corrected)140 {141 intermediateResults.Add(142 new WeightAllowanceCorrection(143 WeightAllowanceCorrectionType.ExceededMaximumAllowedProductsWeight,144 original: range,145 corrected: (calculatedMin, calculatedMax)146 )147 );148 }149150 return corrected;151 }152153 (decimal min, decimal max) ApplySupportedGrossWeightCorrection(154 (decimal min, decimal max) range155 )156 {157 decimal calculatedMin = range.min;158 decimal calculatedMax = range.max;159 calculatedMin = Math.Max(calculatedMin, 0);160 calculatedMin = Math.Min(calculatedMin, MaxSupportedWeightGrams);161 calculatedMax = Math.Max(calculatedMax, 0);162 calculatedMax = Math.Min(calculatedMax, MaxSupportedWeightGrams);163164 var corrected = (calculatedMin, calculatedMax);165166 if (range != corrected)167 {168 intermediateResults.Add(169 new WeightAllowanceCorrection(170 WeightAllowanceCorrectionType.ExceededSupportedGrossWeight,171 original: range,172 corrected: (calculatedMin, calculatedMax)173 )174 );175 }176177 return corrected;178 }179 }180 }181182 private readonly IWeighingSettings _settings;183184 public WeightAllowanceCalculator(IWeighingSettings settings)185 {186 _settings = settings ?? throw new ArgumentNullException(nameof(settings));187 }188 }189}
Packaging machine
This is actual code from the Packaging Machine. Feel free to scroll through it.
Packaging
Sometimes, the product fits in one of our standard boxes of bags and other times, the product is delivered in the sturdy box the manufacturer already used. This has consequences for the path that the order takes in our warehouse.
The weight of the product is unknown
Sometimes, we don't know the weight of the product. For example, because there's wrong product data in our system. These products are marked, so we can measure and weigh them again later.
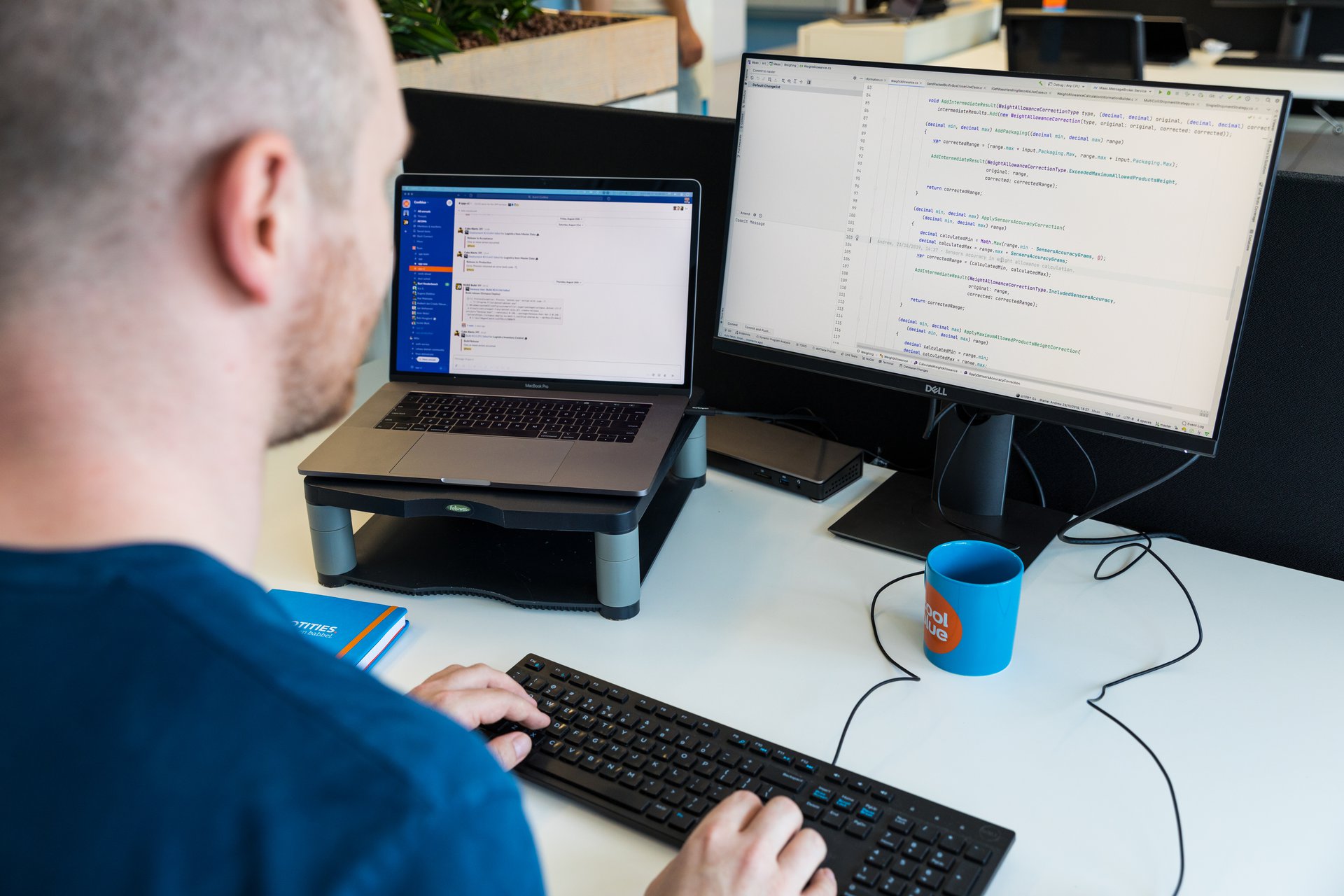
More code
Have another byte